Xamarin iOS - OLD
iOS 14 Implementation
In order to give you full control over the user experience, the Branch SDK will not trigger the IDFA permission modal.
However, we will still collect and use IDFAs when available if you do choose to trigger the modal.
Inconsistent Universal links behavior on iOS 11.2
After updating a device to iOS 11.2, we found that the app's AASA file is no longer downloaded reliably onto your user’s device after an app install. As a result, clicking on Universal links will no longer open the app consistenly. You can set forced uri redirect mode on your Branch links to open the app with URI schemes. View details of the issue on the Apple Bug report.
Configure Branch
- Complete your Branch Dashboard
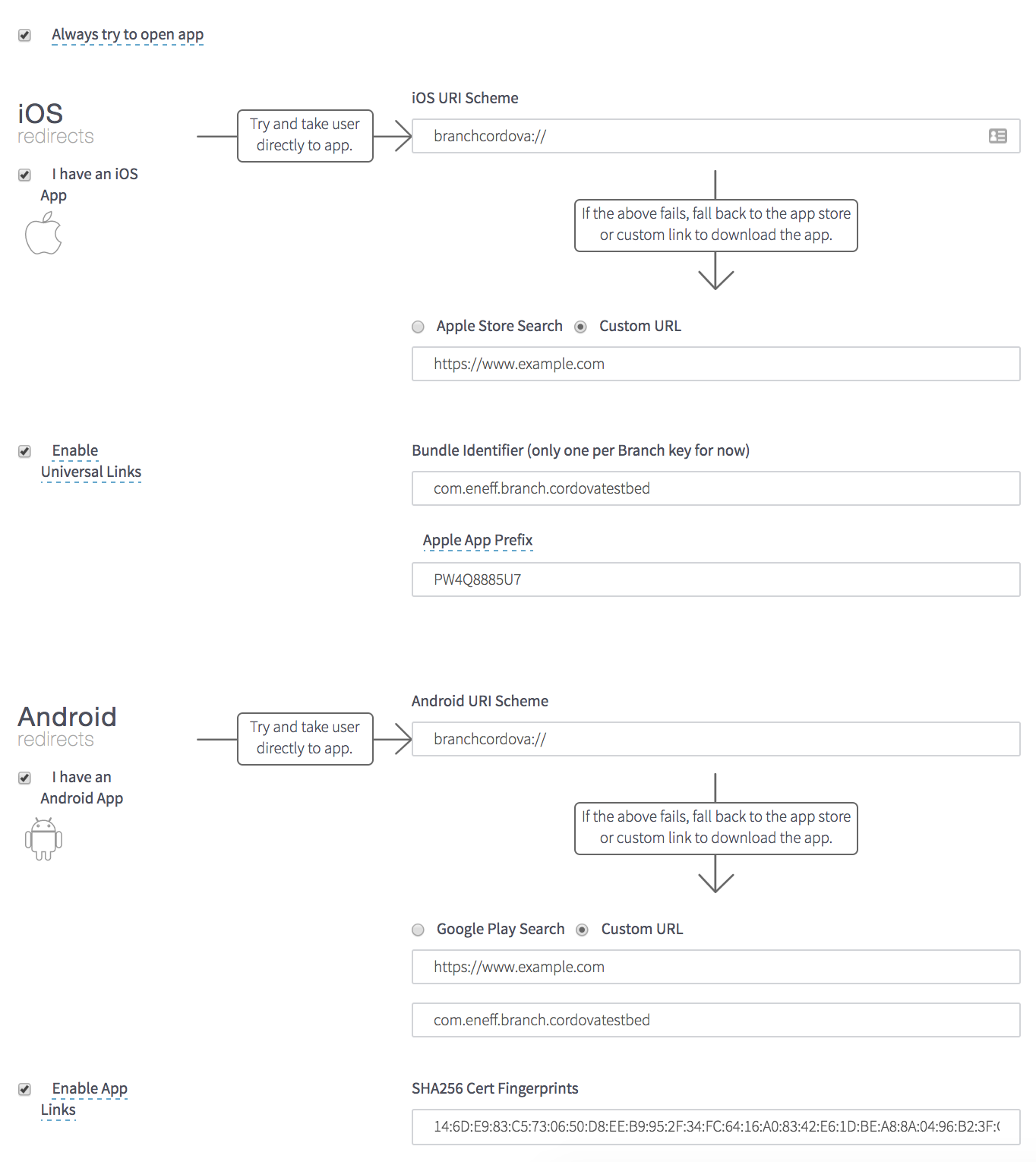

Install Branch
NuGet
The Branch Xamarin SDK is available as a NuGet package. The Branch NuGet package must be added to each of the Xamarin projects that will use Branch methods.
To add the Branch NuGet package to a project:
- Right-click on each project and select Add > Add NuGet Packages
- Find the Branch Xamarin SDK package and add it to the project
Configure App
Create an Apple device Provisioning Profile for the app
- Open Xcode and create a new project with the same name as your Xamarin iOS project
- On the Xcode project's General tab, verify the app's case-sensitive Bundle Identifier is correct and select the appropriate Team (be sure to resolve any errors here)
- Select the Capabilities tab and enable the Associated Domains entitlement
- Create 'applinks:' entries for the Branch link domain and the alternate link domain (the link domain can be found at the bottom of the Branch dashboard's Link Settings page). For example, the entries for an app with the default link domain 'testiosapp.app.link' would be:
applinks:testiosapp.app.link
applinks:testiosapp-alternate.app.link
- Use Xcode to run this newly-created app on an iOS device. This will create and install a Provisioning Profile with the proper entitlements on that device.
- Close Xcode
Enter the app's settings on the Branch dashboard
- On the Link Settings page, check the I have an iOS App checkbox
- Enter the app's URI Scheme in the iOS URI Scheme field (for an app with the URI Scheme testbed-xamarin, for example, the entry would be:
testbed-xamarin://
) - Enter the app's Apple Store name in the Apple Store Search field (if the app is not yet available on the App Store select Custom URL and enter as a placeholder the URL of an appropriate web site - the exact site does not matter)
- Check the Enable Universal Links checkbox
- Enter the app's case-sensitive Bundle Identifier and Apple App Prefix as shown on the Apple Developer Portal
Configure the Xamarin project's Info.plist file
- Open the Info.plist file
- Enter the app's Bundle Identifier from the Apple Developer Portal in the Bundle Identifier field. IMPORTANT: this field will automatically be populated with an all-lowercase value by Xamarin. The value is in fact case sensitive and must match the value in the Apple Developer Portal precisely.
- Click on the Advanced tab
- In the URL Types section, click the Add URL Type button
Identifier: Branch Scheme
URL Schemes: {the app's URI Scheme - 'testiosapp', for example} Role: Editor
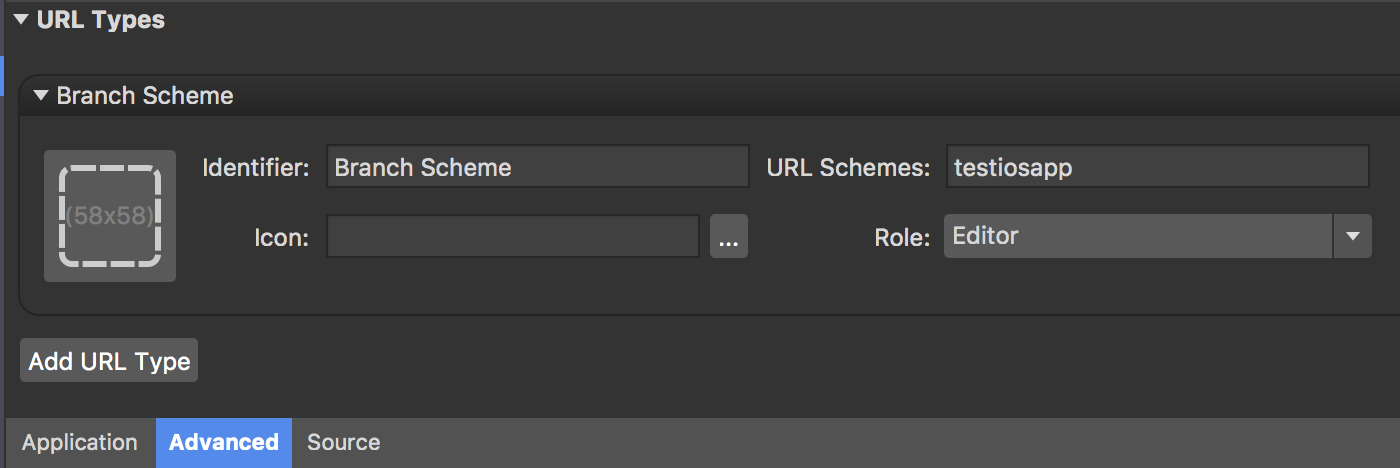
Configure the Xamarin project's Associated Domains entitlement
- Open the Entitlements.plist file and browse to Associated Domains
- Create entries for both the app's link domain and its alternate link domain. The entries for the TestBed-Xamarin app would be:
applinks:testiosapp.app.link
applinks:testiosapp-alternate.app.link
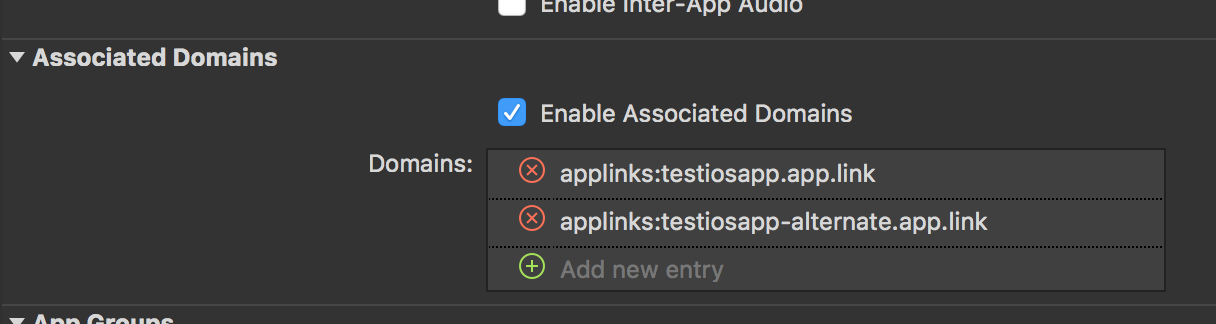
Update the project's Signing Identity and Provisioning Profile
- Right-click on the iOS project and select Options
- Select iOS Bundle Signing
- Set the Signing Identity and Provisioning Profile values to the values used when deploying the Provisioning Profile to the device above
Initialize Branch
Configure Branch with Xamarin Forms
Branch initialization occurs within the FinishedLaunching
method of the AppDelegate.cs file. Branch calls are also required in the OpenUrl
, ContinueUserActivity
, and ReceiveRemoteNotification
methods to ensure that Branch link information is handled properly whenever the app becomes active.
Whenever the app becomes active, the Branch SDK will reach out to the Branch back end to retrieve any available link parameters. If the app became active due to a click on a Branch link, the link data will be returned in the InitSessionComplete method
. This is where any deep link routing logic should reside. Any error in retrieving Branch link data from the back end will returned in the SessionRequestError
method.
// AppDelegate.cs
using Foundation;
using UIKit;
using BranchXamarinSDK;
using BranchXamarinSDK.iOS;
using System;
namespace TestiOSApp.iOS
{
[Register("AppDelegate")]
public class AppDelegate : UIApplicationDelegate, IBranchBUOSessionInterface
{
public override UIWindow Window
{
get;
set;
}
public override bool FinishedLaunching(UIApplication application, NSDictionary launchOptions)
{
// Debug mode - set to 'false' before releasing to production
BranchIOS.Debug = true;
BranchIOS.Init("key_live_cgEguO4UiDJSL4HIyTu85dkkDAdz38ER", launchOptions, this);
return true;
}
// Called when the app is opened via URI scheme
public override bool OpenUrl(UIApplication application, NSUrl url, string sourceApplication, NSObject annotation)
{
return BranchIOS.getInstance().OpenUrl(url);
}
// Called when the app is opened from a Universal Link
public override bool ContinueUserActivity(UIApplication application, NSUserActivity userActivity,
UIApplicationRestorationHandler completionHandler)
{
return BranchIOS.getInstance().ContinueUserActivity(userActivity);
}
// Called when the app receives a push notification
public override void ReceivedRemoteNotification(UIApplication application, NSDictionary userInfo)
{
BranchIOS.getInstance().HandlePushNotification(userInfo);
}
// Called when the Branch initialization is completed
// Put deep-linking logic in this method
public void InitSessionComplete(BranchUniversalObject buo, BranchLinkProperties blp)
{
NSObject[] keys = {
NSObject.FromObject("+is_first_session")
};
NSObject[] values = { NSObject.FromObject(0) };
if (buo.metadata.ContainsKey("+is_first_session"))
{
values[0] = NSObject.FromObject(buo.metadata["+is_first_session"]);
}
NSDictionary nsData = NSDictionary.FromObjectsAndKeys(values, keys);
}
// Called when there is an error initializing Branch
public void SessionRequestError(BranchError error)
{
Console.WriteLine("Branch error: " + error.ErrorCode);
Console.WriteLine(error.ErrorMessage);
}
}
}
Configure Branch with Xamarin Forms
Create a class for Branch session handling
Branch initializes asynchronously, with Branch link parameters being returned following a network call to Branch. If initialization is successful, the InitSessionComplete method will be invoked. If initialization is unsuccessful, the SessionRequestError method will be invoked. Deep link routing logic should be located in the InitSessionComplete method.
- Right-click on the C# project and select Add > New File...
- Select: General > Empty Class
- Rename the file:TestXamarinFormsApp.cs
- Enter the following code (replacing 'TestXamarinFormsApp' with the actual name of the app):
using BranchXamarinSDK;
using System.Collections.Generic;
using System.ComponentModel;
using Xamarin.Forms;
namespace TestXamarinFormsApp
{
public class TestXamarinFormsApp : Application, IBranchSessionInterface
{
public TestXamarinFormsApp()
{
}
#region IBranchSessionInterface implementation
public void InitSessionComplete(Dictionary<string, object> data)
{
}
public void CloseSessionComplete()
{
}
public void SessionRequestError(BranchError error)
{
}
#endregion
}
}
Create a class for handling link data
Branch stores link data in an object referred to as the Branch Universal Object, or BUO.
- Right-click on the C# project and select Add > New File...
- Select: General > Empty Class
- Rename the file: TestXamarinFormsAppBUO.cs
- Enter the following code (replace 'TestXamarinFormsApp' with the actual name of the app):
using BranchXamarinSDK;
using System.Collections.Generic;
using System.ComponentModel;
using Xamarin.Forms;
namespace TestXamarinFormsApp
{
public class TestXamarinFormsAppBUO : Application, IBranchBUOSessionInterface
{
public TestXamarinFormsAppBUO()
{
}
#region IBranchBUOSessionInterface implementation
public void InitSessionComplete(BranchUniversalObject buo, BranchLinkProperties blp)
{
}
public void SessionRequestError(BranchError error)
{
}
#endregion
}
}
Add Branch calls to the AppDelegate.cs file
To ensure that the Branch SDK initializes when the app starts and can retrieve link parameters whenever the app becomes active, Branch initialization occurs within the FinishedLaunching
method of the AppDelegate.cs file. Branch calls are also required in the OpenUrl, ContinueUserActivity, and ReceiveRemoteNotification methods to ensure that Branch link information is handled properly whenever the app becomes active. The AppDelegate.cs file should look like this:
using System;
using System.Collections.Generic;
using System.Linq;
using Foundation;
using UIKit;
using BranchXamarinSDK;
using TestXamarinFormsApp;
namespace TestXamarinFormsApp.iOS
{
[Register("AppDelegate")]
public partial class AppDelegate : global::Xamarin.Forms.Platform.iOS.FormsApplicationDelegate
{
public override bool FinishedLaunching(UIApplication app, NSDictionary options)
{
global::Xamarin.Forms.Forms.Init();
// Debug mode - set to 'false' before releasing to production
BranchIOS.Debug = true;
TestXamarinFormsAppBUO appBUO = new TestXamarinFormsAppBUO();
BranchIOS.Init("key_live_liAnF8k7gZUEZv76Rt9a4bffAzlC5zVW", options, appBUO);
LoadApplication(appBUO);
return base.FinishedLaunching(app, options);
}
// Called when the app is opened via URI scheme
public override bool OpenUrl(UIApplication application, NSUrl url, string sourceApplication, NSObject annotation)
{
return BranchIOS.getInstance().OpenUrl(url);
}
// Called when the app is opened from a Universal Link
public override bool ContinueUserActivity(UIApplication application, NSUserActivity userActivity, UIApplicationRestorationHandler completionHandler)
{
return BranchIOS.getInstance().ContinueUserActivity(userActivity);
}
// Called when the app receives a push notification
public override void ReceivedRemoteNotification(UIApplication application, NSDictionary userInfo)
{
BranchIOS.getInstance().HandlePushNotification(userInfo);
}
}
}
Implement Features
Create content reference
- The Branch Universal Object encapsulates the thing you want to share
- Uses Universal Object properties
BranchUniversalObject universalObject = new BranchUniversalObject();
universalObject.canonicalIdentifier = "id12345";
universalObject.title = "id12345 title";
universalObject.contentDescription = "My awesome piece of content!";
universalObject.imageUrl = "https://s3-us-west-1.amazonaws.com/branchhost/mosaic_og.png";
universalObject.metadata.AddCustomMetadata("foo", "bar");
Create deep link
- Generates a deep link within your app
- Needs a Create content reference
- Needs a Create link reference
- Validate with the Branch Dashboard
BranchLinkProperties linkProperties = new BranchLinkProperties();
linkProperties.tags.Add("tag1");
linkProperties.tags.Add("tag2");
linkProperties.feature = "sharing";
linkProperties.channel = "facebook";
linkProperties.controlParams.Add("$desktop_url", "http://example.com");
Branch.GetInstance().GetShortURL(callback,
universalObject,
linkProperties);
Share deep link
- Will generate a Branch deep link and tag it with the channel the user selects
- Needs a Create content reference
- Needs a [Create link reference]/developers-hub/docs/ios-advanced-features#section-create-link-reference)
- Uses Deep Link Properties
Branch.GetInstance().ShareLink (callback,
universalObject,
linkProperties,
message)
Read deep link
- Retrieve Branch data from a deep link
- Best practice to receive data from the
listener
(to prevent a race condition) - Returns deep link properties
// latest
Dictionary<string, object> sessionParams = Branch.GetInstance().GetLatestReferringParams();
// first
Dictionary<string, object> installParams = Branch.GetInstance().GetFirstReferringParams();
NativeLink™ Deferred Deep Linking (iOS Only)
- Use iOS pasteboard to enable deferred deep linking via Branch NativeLink™
Prerequisites
Make sure you are on v7.1.2+
To use this feature you must:
- Enable NativeLink™ Deep Linking in the Branch Dashboard Configuration tab
or- Manually configure your Branch Link to use
$ios_nativelink
Implement one of the pasteboard opt-in options in the native iOS SDK code.
Track users
- Sets the identity of a user (ID, UUID, etc) for events, deep links, and referrals
- Validate with the Branch Dashboard
Branch branch = Branch.GetInstance ();
branch.SetIdentity("the user id", this); // Where this implements IBranchIdentityInterface
branch.Logout(this); // Where this implements IBranchIdentityInterface
Track commerce events
- Commerce events describe events that relate to a customer interacting with your products and converting by purchasing. These are events like adding payment information, purchasing, viewing products, etc. If you have enabled Branch Universal Ads, these events will automatically map to certain Ad Partners. Start by creating a Branch Universal Object for each product that is associated with the event you're tracking.
- From there, add the Branch Universal Object to the tracked event, and use the right predefined constant. For example, the code snippet below is to track when a user adds to cart, but simply replace that constant with another constant to track a different event.
- A note on currency and exchange rates:
If you track commerce events without a currency, we assume they are USD. If you track commerce events with a currency other than USD, we will convert the revenue specified to USD, using a recent exchange rate. - This allows you to easily visualize revenue on the Dashboard, across many countries and currencies, because all units are USD. The exchange rate is pulled from openexchangerates.org regularly, and is generally within an hour of the realtime exchange rate. If you view raw Branch events via either Webhooks or Exports, you can see the exchange rate used.
- Validate with the Branch Dashboard
BranchUniversalObject universalObject = new BranchUniversalObject();
universalObject.canonicalIdentifier = "id12345";
universalObject.canonicalUrl = "https://branch.io/item/id12345";
universalObject.title = "Item 12345";
universalObject.contentDescription = "My awesome piece of content!";
universalObject.imageUrl = "https://s3-us-west-1.amazonaws.com/branchhost/mosaic_og.png";
universalObject.metadata.price = (float)23.20;
universalObject.metadata.quantity = 1;
universalObject.metadata.sku = "1994329302";
universalObject.metadata.productName = "my_product_name1";
universalObject.metadata.productBrand = "my_product_brand1";
universalObject.metadata.productCategory = BranchProductCategory.APPAREL_AND_ACCESSORIES;
universalObject.metadata.productVariant = "XL";
universalObject.metadata.condition = BranchCondition.NEW;
universalObject.metadata.AddCustomMetadata("foo", "bar");
BranchEvent branchCommerceEvent = new BranchEvent(BranchEventType.PURCHASE);
branchCommerceEvent.SetAlias("new_user_purchase");
branchCommerceEvent.AddContentItem(universalObject);
branchCommerceEvent.SetRevenue((float)1.5);
branchCommerceEvent.SetShipping((float)10.5);
branchCommerceEvent.SetCurrency(BranchCurrencyType.USD);
branchCommerceEvent.SetTax((float)12.3);
Branch.GetInstance().SendEvent(branchCommerceEvent);
Track content events
- Content events are events that occur when a user engages with your in-app content. For example, if you have in-app articles, you would want to track events related to when users search, view content, rate the content, and share. This can apply to a wide variety of in-app content, such as blog posts, music, video, pictures, and e-commerce catalogue items.
- Validate with the Branch Dashboard
BranchEvent branchContentEvent = new BranchEvent(BranchEventType.SEARCH);
branchContentEvent.SetAlias("my_custom_alias");
branchContentEvent.SetDescription("Product Search");
branchContentEvent.SetSearchQuery("user search query terms for product xyz");
branchContentEvent.AddCustomData("content_event_property_key1", "content_event_property_val1");
Branch.GetInstance().SendEvent(branchContentEvent);
Track lifecycle events
- Lifecycle events can be described as events a user takes in your app to continue progressing. These events can apply to game apps, as well as non game apps, for when a user completes a user profile, registration or tutorial.
- Validate with the Branch Dashboard
BranchEvent branchLifecycleEvent = new BranchEvent(BranchEventType.COMPLETE_REGISTRATION);
branchLifecycleEvent.SetAlias("registration_flow_xyz"); branchLifecycleEvent.SetDescription("Existing User Registration");
branchLifecycleEvent.AddCustomData("lifeycle_event_property_key1", "lifecycle_event_property_val1");
Branch.GetInstance().SendEvent(branchLifecycleEvent);
Track custom events
- If you want to track an event that isn't a predefined event, simply do the following:
- We strongly recommend using custom event names that contain no more than 40 characters, contain only letters, numbers, hyphens, spaces and underscores, and do not start with a hyphen. Facebook will not accept events that violate these rules, and if you enable the Facebook integration, Branch may sanitize your event names to pass validation.
- Validate with the Branch Dashboard
BranchEvent branchCustomEvent = new BranchEvent("custom_event");
branchCustomEvent.SetAlias("custom_event_alias");
branchCustomEvent.SetDescription("Custom Event Description");
branchCustomEvent.AddCustomData("custom_event_property_key1", "custom_event_property_val1");
branchCustomEvent.AddCustomData("custom_event_property_key2", "custom_event_property_val2");
Branch.GetInstance().SendEvent(branchCustomEvent);
Troubleshoot Issues
Test deep link iOS
- Create a deep link from the Branch Marketing Dashboard
- Delete your app from the device
- Compile your app with Xcode
- Paste deep link in Apple Notes
- Long press on the deep link (not 3D Touch)
- Click Open in "APP_NAME" to open your app
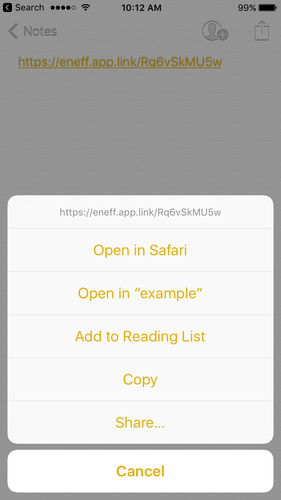
Unable to add Branch-required NuGet packages to the C# project
Adding required NuGet packages to the C# project may fail if the project has not been configured to use PCL 4.5 - Profile78
- Right-click on the project name and select: Options
- Browse the menu to Build > General
- Change the Current Profile to: PCL 4.5 - Profile78
Deploying iOS app to device fails with Provisioning Profile erros after changing entitlements
Xamarin automatically populates the Bundle Identifier field in the Info.plist file with an all-lowercase value derived from the app's name. This value is in fact case sensitive and must match the value in the Apple Developer Portal precisely. The default Xamarin configuration may work when there are no entitlements configured and then suddenly begin failing after entitlements have been added.
This issue can be resolved by ensuring that the Bundle Identifier in the Info.plist matches the Bundle Identifier shown on the Apple Developer Portal.
- Open the Info.plist file
- Enter the app's Bundle Identifier from the Apple Developer Portal in the Bundle Identifier field
Sample apps
There are four fully-functional demo apps included in this repository: a Xamarin Native and Xamarin Forms apps for both iOS and Android platforms. Use these apps as reference models for integrating the Branch SDK.
- Testbed-Xamarin (Native apps)
Testbed-XamarinForms (Forms apps)
- iOS
Updated over 1 year ago