Android Testing
Test Branch functionality and access tools to ensure you've successfully integrated our Android SDK.
Overview
The test scenarios, tools, and resources in this guide help you make sure that you've integrated the Branch Android SDK correctly.
To integrate the Branch Android SDK, follow the steps in our Android SDK Basic Integration guide.
Test Scenarios
Test Deep Linking
To test whether you can successfully use Branch Deep Links:
- Create a deep link using the Branch Dashboard.
- Make sure your test device is ready to use.
- Remove the app from your device and reset your advertising ID.
- Compile and re-install your app on the device.
- Paste the deep link in a different app on the device - somewhere that you'll be able to click the link from.
- Click the deep link. Your app should open and route you to the proper screen.
Test Deep Link Routing
To test deep link routing for your app:
- Append
?bnc_validate=true
to a Branch Deep Link. - Click on this deep link from your mobile device (not the Simulator).
An example link would look like: "https://<yourapp\>.app.link/NdJ6nFzRbK?bnc_validate=true"
Test Install
To ensure the SDK is setup correctly, you no longer need to simulate an install via the SDK itself.
Instead, you can test functionality end to end by completing the following:
- Add a test device to your Branch account.
- Test your campaign setup.
Testing Tools
Integration Status Tab
For a quick approach to checking your Branch Android SDK integration status and progress, you can use the Integration Status tab of the Branch Dashboard.
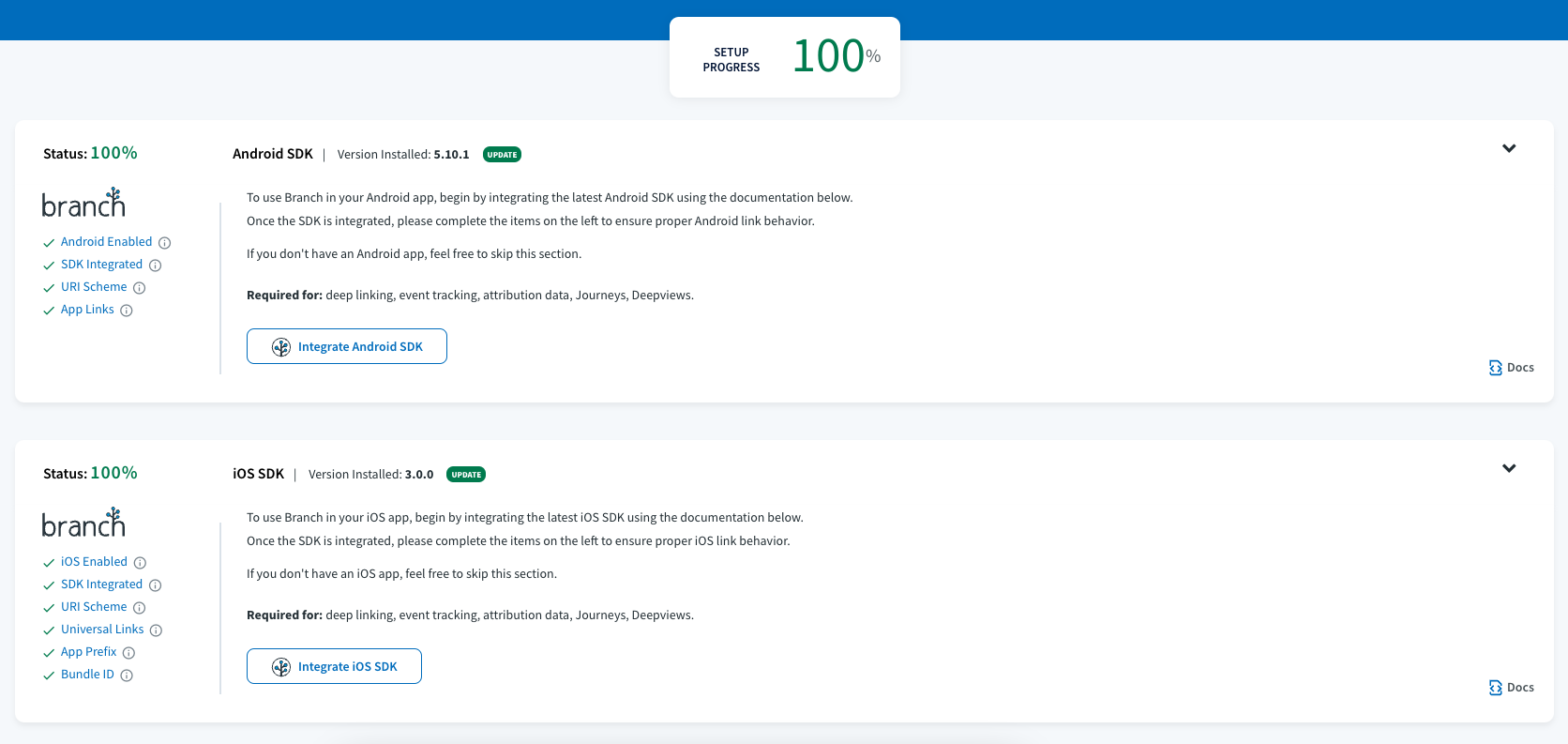
Integration Validator Method
Another simple way to test the status of your Branch Android SDK integration is using the built-in IntegrationValidator.validate()
method.
To use this method, add the following code to your MainActivity's onStart()
:
IntegrationValidator.validate(this)
IntegrationValidator.validate(MainActivity.this);
This method will check to ensure that the Branch keys, package name, URI schemes, and link domain settings from the Branch Dashboard match those in the build.
Check your ADB Logcat to make sure all the SDK integration tests pass.
Make sure to comment out or remove IntegrationValidator.validate()
in your production build.
For more about the IntegrationValidator.validate()
method, visit our blog.
Branch Test Key
For testing purposes, you can use your Branch test key instead of your live key.
To use your test key:
-
Add your test key to your
AndroidManifest.xml
file. -
Use the sample code below to update your app, noting the
enableTestMode()
method:package com.example.android import android.app.Application import io.branch.referral.Branch class CustomApplicationClass : Application() { override fun onCreate() { super.onCreate() // Branch logging for debugging Branch.enableTestMode() // Branch object initialization Branch.getAutoInstance(this) } }
package com.example.android; import android.app.Application; import io.branch.referral.Branch; public class CustomApplicationClass extends Application { @Override public void onCreate() { super.onCreate(); // Branch logging for debugging Branch.enableTestMode(); // Branch object initialization Branch.getAutoInstance(this); } }
Make sure the test key of your app matches the test key in the deep link, and that you remove the enableTestMode()
method before releasing to production.
Enable Logging
Enable logging in your app to catch errors and other useful information coming from Branch. Make sure to only do this for testing, and that you remove the logging code before releasing to production.
Use the sample code below to update your app with any of the enableLogging()
methods:
package com.example.android
import android.app.Application
import io.branch.referral.Branch
class CustomApplicationClass : Application() {
override fun onCreate() {
super.onCreate()
// Branch logging for debugging
Branch.enableLogging()
// Adjust the desired log level (Android SDK versions v5.12.0+ only)
Branch.enableLogging(BranchLogger.BranchLogLevel.VERBOSE)
// Create a custom callback to forward log messages to
val loggingCallbacks = IBranchLoggingCallbacks { logMessage, severityConstantName ->
// Handle the log messages
Log.v("CustomTag", logMessage)
}
Branch.enableLogging(loggingCallbacks)
// Branch object initialization
Branch.getAutoInstance(this)
}
}
package com.example.android;
import android.app.Application;
import io.branch.referral.Branch;
public class CustomApplicationClass extends Application {
@Override
public void onCreate() {
super.onCreate();
// Branch logging for debugging
Branch.enableLogging();
// Adjust the desired log level (Android SDK versions v5.12.0+ only)
Branch.enableLogging(BranchLogger.BranchLogLevel.VERBOSE);
// Create a custom callback to forward log messages to
IBranchLoggingCallbacks loggingCallbacks = new IBranchLoggingCallbacks() {
@Override
public void onBranchLog(String logMessage, String severityConstantName) {
// Handle the log messages
Log.v( "CustomTag", logMessage);
}
};
Branch.enableLogging(loggingCallbacks);
// Branch object initialization
Branch.getAutoInstance(this);
}
}
Link Debugger
You can make sure your Branch Link is properly configured by using Branch's Link Debugger tool. It can help you determine whether the link was properly configured and passed the correct data when it was created.
To use Branch's Link Debugger:
-
Sign in to your Branch Dashboard.
-
Make sure you are in the proper environment for the link you want to debug (live or test).
-
Copy the Branch Link, and append
?debug=1
to the end of it.- For example, the Branch Link
https://branchster.app.link/3vqEJflHrGb
would becomehttps://branchster.app.link/3vqEJflHrGb?debug=1
- For example, the Branch Link
-
Paste this link, including the
?debug=1
flag, into your browser. This will open the Link Debugger view:
This tool includes the Link Routing Debugger, which allows you to view the expected behavior for each operating system and click location.
To use the Link Routing Debugger:
-
Select an operating system and location using the dropdown menus.
-
View the redirect results table for the link.
Based on the operating system and click location you have selected, you’ll see the click redirect outcome for when a user does and does not have the app installed.
Additional Resources
Sample Applications
- Branchsters
- Testbed
- Additional Android SDK sample applications
Troubleshooting Guide
If you're experiencing unexpected behavior with the Branch Android SDK, start by taking a look at our Troubleshooting guide.
Updated 3 months ago